Unityのスクリプトリファレンスです。
https://docs.unity3d.com/ScriptReference/index.html
objectに対するスクリプトを記述していきます。
#pragma strict
function Start () {
Debug.Log("hello world");
}
function Update () {
}
unityのJSは変数の宣言など書き方が少し異なります。
#pragma strict
var x : int = 5;
function Start () {
Debug.Log("hello world ->" + x);
}
function Update () {
}
オブジェクトを動かす際には、transformのポジションを動かします。
function Update () {
transform.position.z += 0.1;
}
vector3でオブジェクトを移動させることもできます。
function Update () {
transform.position += Vector3(0, 0, 0.1);
}
ユーザの入力受付
function Update () {
if (Input.GetButtonUp("Jump")){
Debug.Log("Jumped!");
}
}
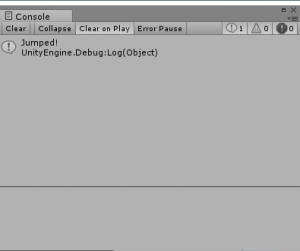
Edit->ProjectSetting->Inspectorで値を参照できます。
左右キーでオブジェクトを移動させます。
function Update () {
if (Input.GetButtonUp("Jump")){
Debug.Log("Jumped!");
}
var x : float = Input.GetAxis("Horizontal");
transform.Translate(x * 0.2, 0, 0);
}
Digitbodyを設定して、以下のように記載すると、衝突判定ができます。
#pragma strict
function Start () {
}
function Update () {
if (Input.GetButtonUp("Jump")){
Debug.Log("Jumped!");
}
var x : float = Input.GetAxis("Horizontal");
transform.Translate(x * 0.2, 0, 0);
}
function OnCollisionEnter(){
Debug.Log("Hit!");
}
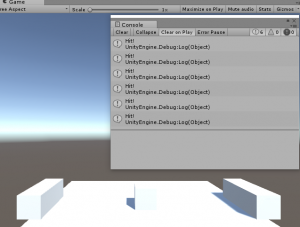
#pragma strict
var ball : Transform;
function Update () {
if (Input.GetButtonUp("Jump")){
Instantiate(ball, transform.position, transform.rotation);
}
}