Laravelでseleniumを使おうと思ったら、duskというパッケージがあるらしいので、duskをインストールして使っていきます。環境は、vagrant、amazon linux2, laravel 7.2.1です。
### 1.duskインストール
$ php composer.phar require –dev laravel/dusk
– laravel/dusk v5.10.0 requires ext-zip * -> the requested PHP extension zip is missing from your system.
$ sudo yum install php-pecl-zip.x86_64
$ php composer.phar require –dev laravel/dusk
$ php artisan dusk:install
testsディレクトリにBrowserディレクトリが作られる
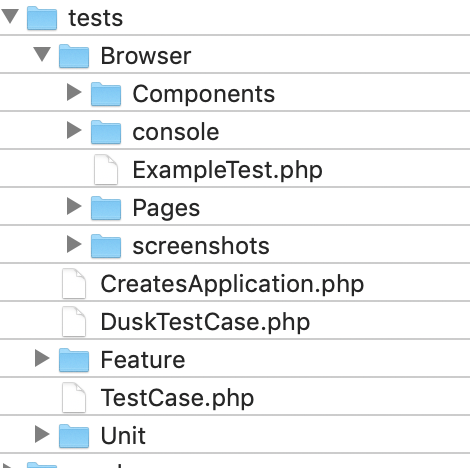
.env
APP_URL=http://192.168.33.10:8000
### 2.duskテスト
$ php artisan dusk:make LoginTest
/tests/Browser/LoginTest.php
public function test_can_login_successfull(){
$this->browse(function (Browser $browser) {
$browser->visit('/login')
->type('email', 'foo@hoge.com')
->type('password', 'hogehoge')
->press('Login')
->assertSee('this is subscriber');
});
}
$ php artisan dusk
1) Tests\Browser\ExampleTest::testBasicExample
Facebook\WebDriver\Exception\WebDriverCurlException: Curl error thrown for http POST to /session with params: {"capabilities":{"firstMatch":[{"browserName":"chrome","goog:chromeOptions":{"binary":"","args":["--disable-gpu","--headless","--window-size=1920,1080"]}}]},"desiredCapabilities":{"browserName":"chrome","platform":"ANY","chromeOptions":{"binary":"","args":["--disable-gpu","--headless","--window-size=1920,1080"]}}}
Failed to connect to localhost port 9515: Connection refused
ん? ChromeDriverが入っていない?
### 3.ChromeDriver インストール
$ ./vendor/laravel/dusk/bin/chromedriver-linux –verbose
./vendor/laravel/dusk/bin/chromedriver-linux: error while loading shared libraries: libX11.so.6: cannot open shared object file: No such file or directory
$ sudo yum install -y libX11
$ ./vendor/laravel/dusk/bin/chromedriver-linux –v
ChromeDriver 80.0.3987.106 (f68069574609230cf9b635cd784cfb1bf81bb53a-refs/branch-heads/3987@{#882})
$ php artisan dusk
1) Tests\Browser\ExampleTest::testBasicExample
Facebook\WebDriver\Exception\UnknownErrorException: unknown error: cannot find Chrome binary
なに、今度はChrome binary?
### 4.Chrome install
$ curl https://intoli.com/install-google-chrome.sh | bash
$ php artisan dusk
1) Tests\Browser\LoginTest::test_can_login_successfull
Facebook\WebDriver\Exception\NoSuchElementException: no such element: Unable to locate element: {"method":"css selector","selector":"body textarea[name='email']"}
(Session info: headless chrome=80.0.3987.149)
どういうこと?
### 5.visitでパスを直接指定
public function test_can_login_successfull(){
$this->browse(function (Browser $browser) {
$browser->visit('http://192.168.33.10:8000/login')
// ->dump();
->type('email', 'foo@hoge.com')
->type('password', 'hogehoge')
->press('Login')
->assertSee('this is subscriber');
});
}
$ php artisan dusk
PHPUnit 8.5.2 by Sebastian Bergmann and contributors.
. 1 / 1 (100%)
Time: 2.18 seconds, Memory: 18.00 MB
OK (1 test, 1 assertion)
上手くいきました。半日かかりましたが、seleniumが何やってるかは理解できました。