Gensim: 自然言語処理ライブラリ、fastTextを実行できる、トピックモデルの作成、tf-idf、Word2Vecなど
$ sudo pip3 install gensim
### 分かち書きした日本語のテキストを用意
$ wget https://s3-ap-northeast-1.amazonaws.com/dev.tech-sketch.jp/chakki/public/ja.text8.zip
$ unzip ja.text8.zip
※small (100MB) text corpus from the wikipedia
https://github.com/Hironsan/ja.text8
$ which fasttext
$ /usr/local/bin/fasttext skipgram -input ja.text8 -output ja.text8.model
一体なんやこれは?????
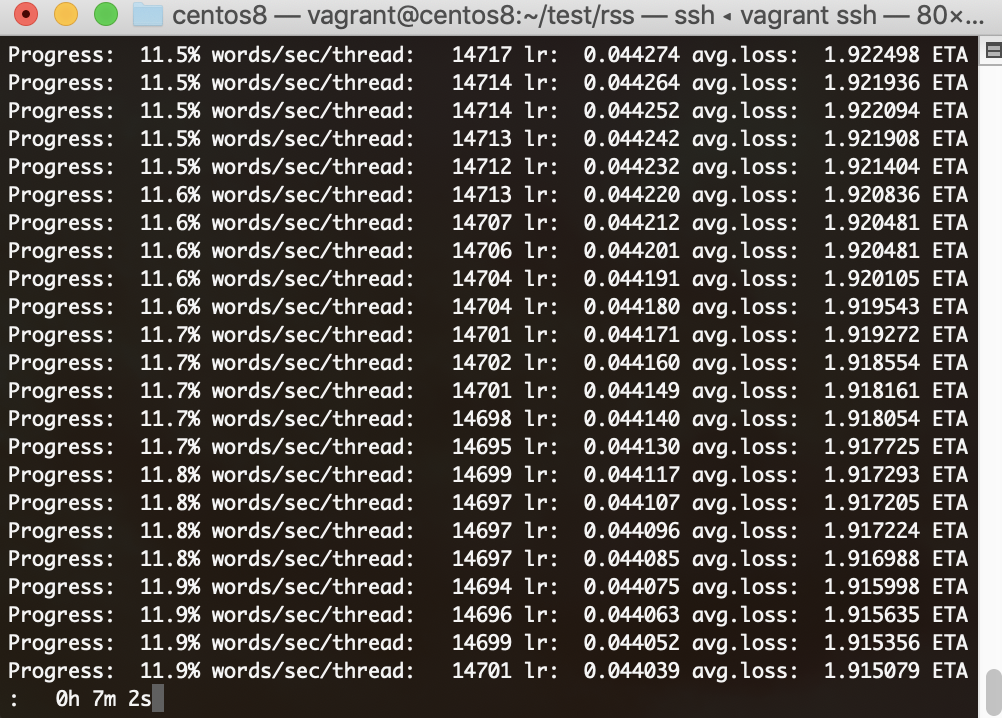
$ ls
app.py ja.text8 ja.text8.model.bin ja.text8.model.vec ja.text8.zip
### 学習済みモデル
$ sudo pip3 install python-Levenshtein
$ less -S ja.text8.model.vec
$ python3
>>> from gensim.models import KeyedVectors
>>> word_vecs = KeyedVectors.load_word2vec_format(‘~/test/rss/ja.text8.model.vec’, binary=False)
>>> word_vecs.most_similar(positive=[‘公園’])
[(‘東公園’, 0.7657707929611206), (‘森林公園’, 0.7654288411140442), (‘園内’, 0.7647179961204529), (‘西公園’, 0.7584214210510254), (‘レクリエーション’, 0.7228037118911743), (‘緑地公園’, 0.7194845676422119), (‘遊歩道’, 0.7175000905990601), (‘芝公園’, 0.7167261242866516), (‘グチ’, 0.7141640186309814), (‘花瀬’, 0.7108176946640015)]
>>> word_vecs.most_similar(positive=[‘国王’, ‘女’], negative=[‘男’])
[(‘王妃’, 0.6794450879096985), (‘アルフォンソ’, 0.677681028842926), (‘王族’, 0.6705313324928284), (‘テオドラ’, 0.6641644239425659), (‘アフォンソ’, 0.6623624563217163), (‘エリザヴェータ’, 0.6613386273384094), (‘シャルマネセル’, 0.6583849787712097), (‘王’, 0.6572801470756531), (‘ナバラ’, 0.6571141481399536), (‘アブデュルハミト’, 0.6528143882751465)]
>>> word_vecs.most_similar(positive=[‘駅’], negative=[])
[(‘紀勢本線’, 0.829847514629364), (‘信越本線’, 0.8256024122238159), (‘南海本線’, 0.8238951563835144), (‘東上本線’, 0.8169854879379272), (‘羽越本線’, 0.8133241534233093), (‘本四備讃線’, 0.8125778436660767), (‘中央本線’, 0.8098829984664917), (‘総武本線’, 0.8089748024940491), (‘関西本線’, 0.8083009719848633), (‘日豊本線’, 0.8078861832618713)]
つまりKeyedVectorsでmost_similarで類語を検出したり、単語の計算ができる
>>> word_vecs.most_similar(positive=[‘イチロー’, ‘サッカー’], negative=[‘野球’])[(‘フェイエノールト’, 0.7010015845298767), (‘gk’, 0.6880401372909546), (‘ベンフィカ’, 0.6829041242599487), (‘アウストリア・ウィーン’, 0.6794724464416504), (‘コパ・デル・レイ’, 0.6772794127464294), (‘ガンバ’, 0.6750086545944214), (‘ゴールキーパー’, 0.6735791563987732), (‘ゴールイン’, 0.6722267866134644), (‘ネッツァー’, 0.6708939671516418), (‘ジュビロ’, 0.668694257736206)]
が、学習データによって精度に問題がある。
プロセスとしては、
1. 形態素分析
2. fasttext skipgram -input ja.text8 -output ja.text8.model で単語をベクトルに変更
3. KeyedVectorsで学習済み日本語モデルを読み込んで使用
重み付けとは要するに多数決の理論
機械学習だとどうしてもデータセットが適切か、データ量は十分か、果たしてそのモデルが優れているのか?など、品質を上げる為には自問自答が続く。
そして、ある課題に対し、どのモデルが最適かを提案するには、モデルの構造に関する知識が不可欠であり、特徴を整理しなければならない。
突き詰めると、前頭葉を中心とした人間の脳の機能を理解して、人間が行なっている判断に類するものをモデルに反映させる
文章を読むだけでは理解できない、実際に応用して初めて物になる。
言い換えが必要なシーンを考えるか。考えることが違うな。