– authのユーザ登録(/register)の項目をカスタマイズする
–> デフォルトのカラムに対し、usersテーブルにrole_id(admin/subscriber)が追加されており、authのユーザ登録(/register)でも、role_idのradioボタンを追加して登録できるようにしたい
### usersテーブル
Schema::create('users', function (Blueprint $table) {
$table->bigIncrements('id');
$table->integer('role_id')->nullable();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
### register.blade.php
/resources/views/auth/register.blade.php
<div class="card-body">
<form method="POST" action="{{ route('register') }}">
@csrf
// 省略
<div class="col-md-6">
<div class="form-inline">
<div class="form-check">
<input id="role_id" type="radio" class="form-control mr-1 @error('role_id') is-invalid @enderror" name="role_id" value="1" required autocomplete="off" checked>
<label class="form-check-label mr-3" type="radio">Administrator</label>
</div>
<div class="form-check">
<input id="role_id" type="radio" class="form-control mr-1 @error('role_id') is-invalid @enderror" name="role_id" value="2" required autocomplete="off">
<label class="form-check-label mr-3" type="radio">Subscriber</label>
</div>
</div>
@error('role_id')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
// 省略
</form>
</div>
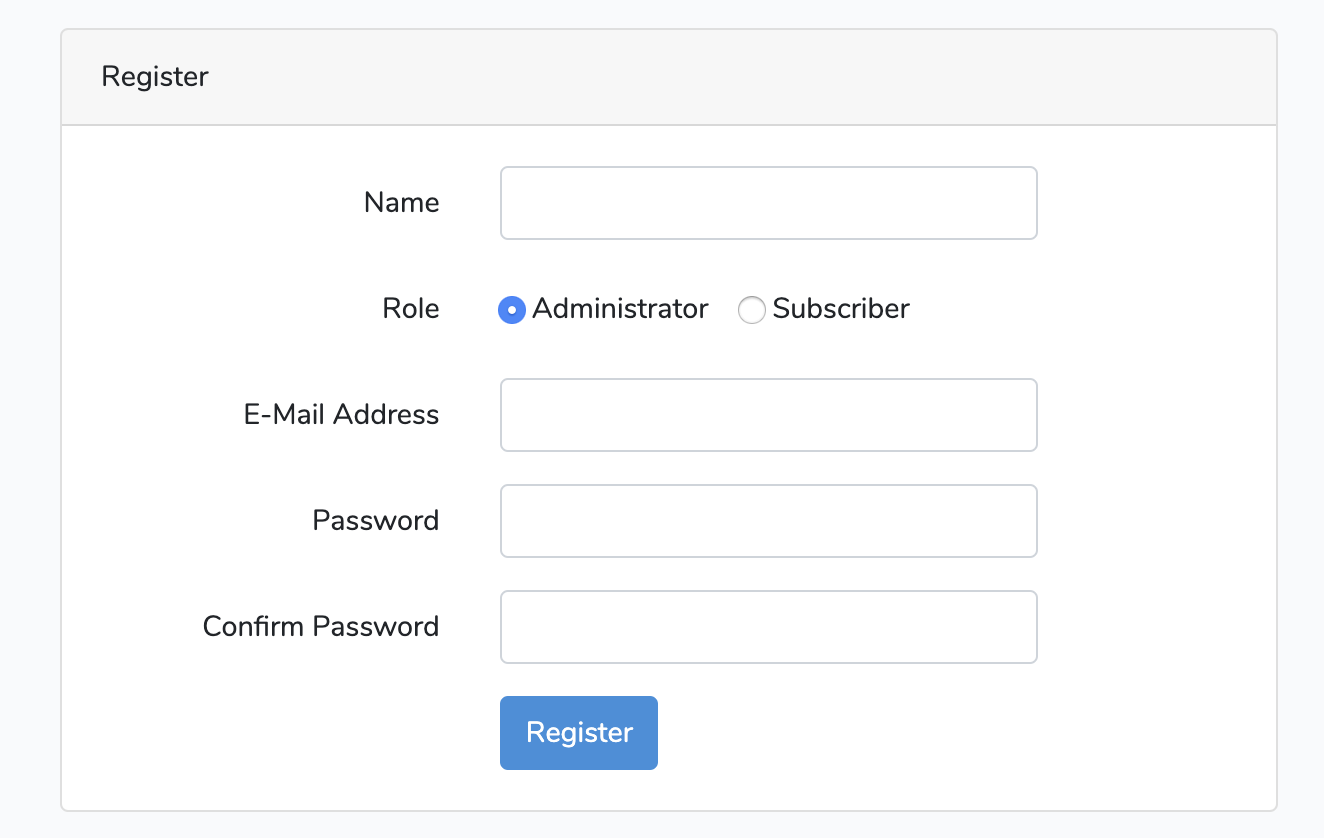
### RegisterController.php
app/Http/Controllers/Auth/RegisterController.php
protected function validator(array $data)
{
return Validator::make($data, [
'name' => ['required', 'string', 'max:255'],
'role_id' => ['required'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
'password' => ['required', 'string', 'min:8', 'confirmed'],
]);
}
/**
* Create a new user instance after a valid registration.
*
* @param array $data
* @return \App\User
*/
protected function create(array $data)
{
return User::create([
'name' => $data['name'],
'role_id' => $data['role_id'],
'email' => $data['email'],
'password' => Hash::make($data['password']),
]);
}
### User.php
protected $fillable = [
'name', 'email', 'role_id', 'password',
];
mysql> select * from users;
$dataとしてPOSTされたデータをcreateしている。
よく編集されるページなのか、RegisterController.phpは非常に分かりやすい構造になっています。