NYダウとドル円の前日比の動きを受けて、翌日の日経がどのように変化したかを見たいと思います。
ダウ、ドル円、日経の前日比の時系列データを用意します。
ここでは、2/1から、2/9まで。
ダウ
日付、始値、高値、安値、終値、前日比、前日比%
2018年2月10日 24,190.9 330.44 +1.38% 23,360.289 24,382.138 735,033,934
2018年2月9日 23,860.458 – -4.15% 23,849.228 24,903.679 657,504,273
2018年2月8日 24,893.349 -19.42 -0.08% 24,785.439 25,293.96 504,623,230
2018年2月7日 24,912.771 567.02 +2.33% 23,778.738 24,946.23 823,936,440
2018年2月6日 24,345.751 – -4.6% 23,923.88 25,520.531 714,449,396
2018年2月3日 25,520.96 -665.75 -2.54% 25,490.66 26,061.791 522,877,741
2018年2月2日 26,186.708 37.32 +0.14% 26,014.439 26,306.701 410,622,631
2018年2月1日 26,149.39 72.50 +0.28% 26,050.978 26,338.029 471,203,651
ドル円
日付、始値、高値、安値、終値、前日比、前日比%
18/02/13 108.60 108.79 107.42 107.80 -0.99 -0.9 0
18/02/09 108.75 109.30 108.05 108.79 +0.06 +0.1 0
18/02/08 109.59 109.78 108.59 108.73 -0.88 -0.8 0
18/02/07 109.55 109.72 108.92 109.61 +0.04 0.0 0
18/02/06 109.23 109.65 108.45 109.57 +0.27 +0.2 0
18/02/05 110.10 110.29 109.14 109.30 -0.83 -0.8 0
18/02/02 109.29 110.48 109.25 110.13 +0.86 +0.8 0
18/02/01 109.17 109.75 109.11 109.27 +0.10 +0.1 0
日経平均
日付、始値、高値、安値、終値、前日比、前日比%
18/02/13 21,633.34 21,679.20 21,211.53 21,244.68 -137.94 -0.6 1,962,390,000
18/02/09 21,507.74 21,510.30 21,119.01 21,382.62 -508.24 -2.3 2,137,480,000
18/02/08 21,721.57 21,977.03 21,649.70 21,890.86 +245.49 +1.1 1,820,420,000
18/02/07 22,001.29 22,353.87 21,627.13 21,645.37 +35.13 +0.2 2,336,290,000
18/02/06 22,267.00 22,277.45 21,078.71 21,610.24 -1,071.84 -4.7 3,155,710,000
18/02/05 22,921.16 22,967.69 22,659.43 22,682.08 -592.45 -2.5 1,881,890,000
18/02/02 23,361.67 23,367.96 23,122.45 23,274.53 -211.58 -0.9 1,702,440,000
18/02/01 23,276.10 23,492.77 23,211.12 23,486.11 +387.82 +1.7
それで、NYダウが+1.38%、ドル円が-0.9動いたときに、翌日nikkeiがどうなるかKNearestNeighborsで算出
<?php
require_once 'vendor/autoload.php';
use Phpml\Classification\KNearestNeighbors;
/*
ダウ
2018年2月10日 24,190.9 330.44 +1.38% 23,360.289 24,382.138 735,033,934
2018年2月9日 23,860.458 - -4.15% 23,849.228 24,903.679 657,504,273
2018年2月8日 24,893.349 -19.42 -0.08% 24,785.439 25,293.96 504,623,230
2018年2月7日 24,912.771 567.02 +2.33% 23,778.738 24,946.23 823,936,440
2018年2月6日 24,345.751 - -4.6% 23,923.88 25,520.531 714,449,396
2018年2月3日 25,520.96 -665.75 -2.54% 25,490.66 26,061.791 522,877,741
2018年2月2日 26,186.708 37.32 +0.14% 26,014.439 26,306.701 410,622,631
2018年2月1日 26,149.39 72.50 +0.28% 26,050.978 26,338.029 471,203,651
ドル円
日付、始値、高値、安値、終値、前日比、前日比%
18/02/13 108.60 108.79 107.42 107.80 -0.99 -0.9 0
18/02/09 108.75 109.30 108.05 108.79 +0.06 +0.1 0
18/02/08 109.59 109.78 108.59 108.73 -0.88 -0.8 0
18/02/07 109.55 109.72 108.92 109.61 +0.04 0.0 0
18/02/06 109.23 109.65 108.45 109.57 +0.27 +0.2 0
18/02/05 110.10 110.29 109.14 109.30 -0.83 -0.8 0
18/02/02 109.29 110.48 109.25 110.13 +0.86 +0.8 0
18/02/01 109.17 109.75 109.11 109.27 +0.10 +0.1 0
日経平均
日付、始値、高値、安値、終値、前日比、前日比%
18/02/13 21,633.34 21,679.20 21,211.53 21,244.68 -137.94 -0.6 1,962,390,000
18/02/09 21,507.74 21,510.30 21,119.01 21,382.62 -508.24 -2.3 2,137,480,000
18/02/08 21,721.57 21,977.03 21,649.70 21,890.86 +245.49 +1.1 1,820,420,000
18/02/07 22,001.29 22,353.87 21,627.13 21,645.37 +35.13 +0.2 2,336,290,000
18/02/06 22,267.00 22,277.45 21,078.71 21,610.24 -1,071.84 -4.7 3,155,710,000
18/02/05 22,921.16 22,967.69 22,659.43 22,682.08 -592.45 -2.5 1,881,890,000
18/02/02 23,361.67 23,367.96 23,122.45 23,274.53 -211.58 -0.9 1,702,440,000
18/02/01 23,276.10 23,492.77 23,211.12 23,486.11 +387.82 +1.7
*/
$dow_usyen = [[0.28, 0.1],[0.14, 0.86],[-2.54, -0.8],[-4.6, 0.2],[2.33, 0],[-0.08, 0.8],[4.15,0.1]];
$nikkei = ['-0.9', '-2.5', '-4.7', '0.2', '1.1', '-2.3','-0.6'];
$classifier = new KNearestNeighbors();
$classifier->train($dow_usyen, $nikkei);
echo $classifier->predict([1.38, -0.9]);
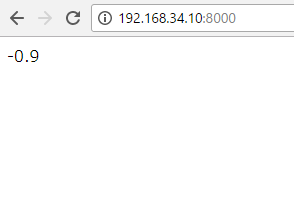
アルゴリズムでは、-0.9が近いと言っています。。。
なにこれ!