モデルにはobjects属性があり、その中にManagerというクラスのインスタンスが入っている
このManagerにfilter機能がある
/hello/urls.py
urlpatterns = [
path('', views.index, name='index'),
path('create', views.create, name='create'),
path('edit/<int:num>', views.edit, name='edit'),
path('delete/<int:num>', views.delete, name='delete'),
path('list', FriendList.as_view()),
path('detail/<int:pk>', FriendDetail.as_view()),
path('find', view.find, name='find'), # 追加
]
/hello/forms.py
class FindForm(forms.Form):
find = forms.CharField(label='Find', required=False, widget=forms.TextInput(attrs={'class':'form-control'}))
/hello/templates/hello/find.html
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<p>{{message|safe}}</p>
<form action="{% url 'find' %}" method="post">
{% csrf_token %}
{{ form.as_p }}
<tr>
<th></th><td><input type="submit" value="click" class="btn btn-primary mt-2"></td>
</tr>
</form>
<table class="table">
<tr>
<th>id</th>
<th>name</th>
<th>mail</th>
</tr>
{% for item in data %}
<tr>
<td>{{item.id}}</td>
<td>{{item.name}}({{item.age}})</td>
<td>{{item.mail}}</td>
</tr>
{% endfor %}
</table>
</body>
/hello/views.py
def find(request):
if(request.method == 'POST'):
form = FindForm(request.POST)
find = request.POST['find']
data = Friend.objects.filter(name=find)
msg = 'Result: ' + str(data.count())
else:
msg = 'search words...'
form = FindForm()
data =Friend.objects.all()
params = {
'title': 'Hello',
'message': msg,
'form': form,
'data': data,
}
return render(request, 'hello/find.html', params)
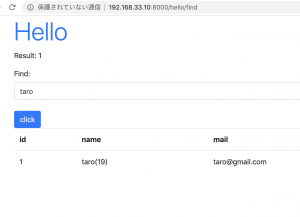
Friend.objects.filter(name=find)で指定している。
### 曖昧検索
– 値を含む検索: __contains=value
– 値で始まるもの: __startswith=value
– 値で終わるもの: __endswith=value
if(request.method == 'POST'):
form = FindForm(request.POST)
find = request.POST['find']
data = Friend.objects.filter(name__contains=find)
msg = 'Result: ' + str(data.count())
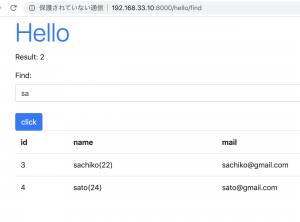
– 大文字小文字を区別しない: __iexact=value
– 曖昧検索: __icontains=value, __istartswith=value, __iendswith=value
### 数値の比較
– 等しい: =value
– 大きい: __gt=value
– 以上: __gte=value
– 小さい: __lt=value
– 以下: __lte=value
if(request.method == 'POST'):
form = FindForm(request.POST)
find = request.POST['find']
data = Friend.objects.filter(age__lte=int(find))
msg = 'Result: ' + str(data.count())
●●以上●●以下
split()は改行やスペースで分割する
if(request.method == 'POST'):
form = FindForm(request.POST)
find = request.POST['find']
val = find.split()
data = Friend.objects.filter(age__gte=val[0], age__lte=val[1])
msg = 'Result: ' + str(data.count())
### AとBどちらも検索
変数 = <<モデル>>.object.filter(Q(条件) | Q(条件))
from django.db.models import Q
//
data = Friend.objects.filter(Q(name__contains=find)|Q(mail__contains=find))
### リストを使って検索
if(request.method == 'POST'):
form = FindForm(request.POST)
find = request.POST['find']
list = find.split()
data = Friend.objects.filter(name__in=list)
msg = 'Result: ' + str(data.count())
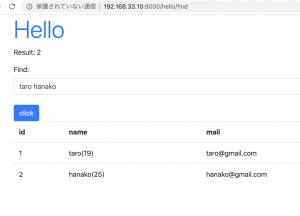
検索条件はアプリケーションの要件や機能によって変わってきますね。