appendとremove
<script src="https://d3js.org/d3.v4.min.js"></script>
<script>
d3.select("body").append("p").text("hello 4").remove();
</script>
値の代入
var dataset = [12, 24, 36];
var p = d3.select("body").selectAll("p");
p.data(dataset).text(function(d, i){
return i + 1 + "番目は" + d + "です!";
});
update, enter
var dataset = [12, 24, 36, 48];
var p = d3.select("body").selectAll("p");
var update = p.data(dataset);
var enter = update.enter();
update.text(function(d){
return "update: " + d;
});
enter.append("p").text(function(d){
return "enter:" + d;
});
棒グラフ
<script src="https://d3js.org/d3.v3.min.js" charset="utf-8"></script>
<script>
var dataset = [11, 25, 45, 30, 33];
var w = 500;
var h = 200;
var svg = d3.select("body").append("svg").attr({width:w, height:h});
svg.selectAll("rect")
.data(dataset)
.enter()
.append("rect")
.attr({
x:0,
y: function(d, i){ return i * 25; },
width: function(d){ return d;},
height: 20,
fill: "red"
});
</script>
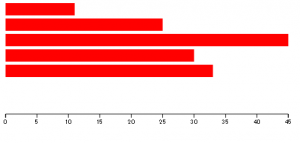
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>D3.js</title>
<style>
.axis path, .axis line{
fill: none;
stroke: black;
}
.axis text {
font-size: 11px;
}
</style>
</head>
<body>
<script src="https://d3js.org/d3.v3.min.js" charset="utf-8"></script>
<script>
var dataset = [11, 25, 45, 30, 33];
var w = 500;
var h = 200;
var padding = 20;
var xScale = d3.scale.linear()
.domain([0, d3.max(dataset)])
.range([padding, w - padding])
.nice();
var svg = d3.select("body").append("svg").attr({width:w, height:h});
var xAxis = d3.svg.axis()
.scale(xScale)
.orient("bottom");
svg.append("g")
.attr({
class: "axis",
transform: "translate(0, 180)"
})
.call(xAxis);
svg.selectAll("rect")
.data(dataset)
.enter()
.append("rect")
.attr({
x:padding,
y: function(d, i){ return i * 25; },
width: function(d){ return xScale(d) - padding;},
height: 20,
fill: "red"
});
</script>
</body>
</html>