/var/www/cgi-bin
[ec2-user@ cgi-bin]$ ls
hello.cgi
[ec2-user@ cgi-bin]$ sudo chmod +x hello.cgi
#!/usr/bin/perl --
print "Content-type: text/html \n\n";
print "Hello";
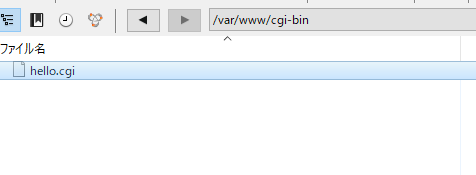
きた

mysql
mysql> create database perldb;
Query OK, 1 row affected (0.02 sec)
mysql> use perldb;
Database changed
mysql> create table t1(
-> a int,
-> b varchar(10)
-> );
Query OK, 0 rows affected (0.04 sec)
mysql> insert into t1 values(1, ‘asakura’),(2, ‘adachi’),(3, ‘kobayakawa’);
Query OK, 3 rows affected (0.00 sec)
Records: 3 Duplicates: 0 Warnings: 0
mysql> select * from t1;
+——+————+
| a | b |
+——+————+
| 1 | asakura |
| 2 | adachi |
| 3 | kobayakawa |
+——+————+
3 rows in set (0.01 sec)
$ perl hoge.pl
Can’t locate DBI.pm in @INC (@INC contains: /usr/local/lib64/perl5 /usr/local/share/perl5 /usr/lib64/perl5/vendor_perl /usr/share/perl5/vendor_perl /usr/lib64/perl5 /usr/share/perl5 .) at hoge.pl line 4.
BEGIN failed–compilation aborted at hoge.pl line 4.
sudo yum -y install perl-DBI perl-DBD-MySQL
きた!OKKKKKKKKKKKKKKKK
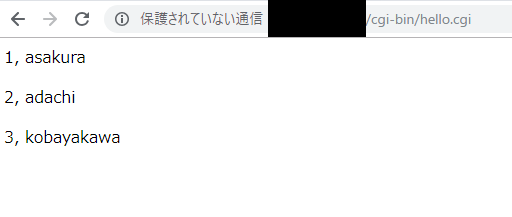
次はservlet mysqlだ。ここわなー