import React, {Component} from 'react';
import Rect from "./Rect";
import './App.css';
class App extends Component {
data = [];
msgStyle = {
fontSize:"20pt",
color:"#900",
margin:"20px 0px",
padding: "5px",
}
area = {
width: "500px",
height: "500px",
border: "1px solid blue"
}
constructor(props) {
super(props);
this.state = {
list: this.data
};
this.doAction = this.doAction.bind(this);
}
doAction(e) {
let x = e.pageX;
let y = e.pageY;
this.data.push({x:x, y:y});
this.setState({
list:this.data
});
}
draw(d) {
let s = {
position:"absolute",
left: (d.x - 25) + "px",
top:(d.y - 25) + "px",
width: "50px",
height: "50px",
backgroundColor: "#66f3",
};
return <div style={s}></div>
}
render() {
return <div className="App">
<h1>React</h1>
<h2 style={this.msgStyle}>show rect. </h2>
<div style={this.area} onClick={this.doAction}>
{this.data.map((value) => this.draw(value))}
</div>
</div>;
}
}
export default App;
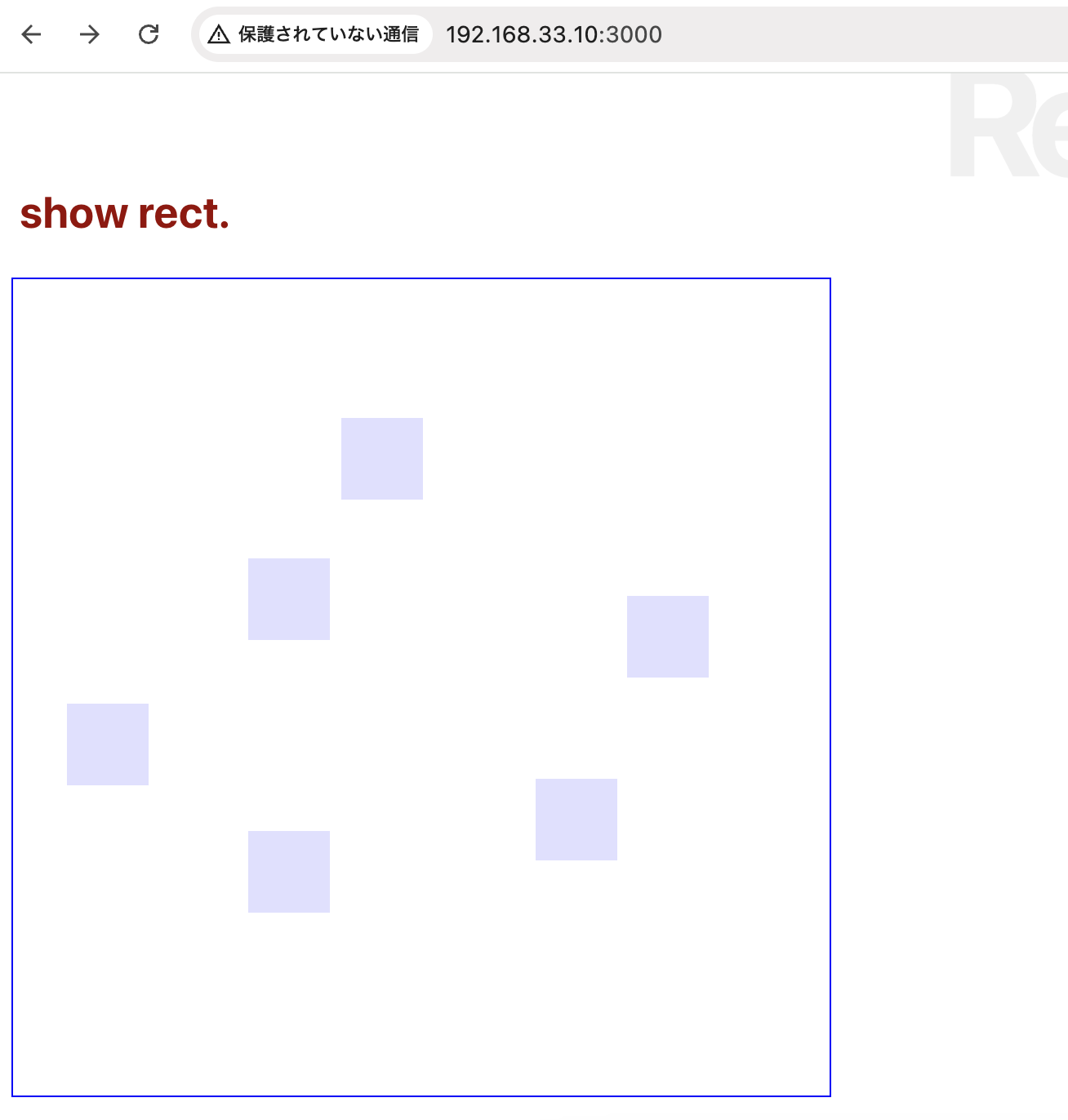
import React, {Component} from 'react';
import Rect from "./Rect";
import './App.css';
class App extends Component {
data = [
"This is list sample",
"これはリストのサンプルです。",
"配列をリストに変換します。"
];
msgStyle = {
fontSize:"20pt",
color:"#900",
margin:"20px 0px",
padding: "5px",
}
constructor(props) {
super(props);
this.state = {
list: this.data
};
}
render() {
return <div className="App">
<h1>React</h1>
<h2 style={this.msgStyle}>show list.</h2>
<List title="サンプル・リスト" data={this.data} />
</div>;
}
}
class List extends Component {
number = 1;
title = {
fontSize:"20pt",
fontWeight:"bold",
color:"blue",
};
render() {
let data = this.props.data;
return (
<div>
<p style={this.title}>{this.props.title}</p>
<ul>
{data.map((item) =>
<Item number={this.number++} value={item} key={this.number} />
)}
</ul>
</div>
);
}
}
class Item extends Component {
li = {
listStyleType:"square",
fontSize:"16pt",
color:"#06",
margin:"0px",
padding: "0px",
}
num = {
fontWeight:"bold",
color:"red"
}
render() {
return (
<li style={this.li}>
<span style={this.num}>[{this.props.number}]</span>
{this.props.value}
</li>
)
}
}
export default App;
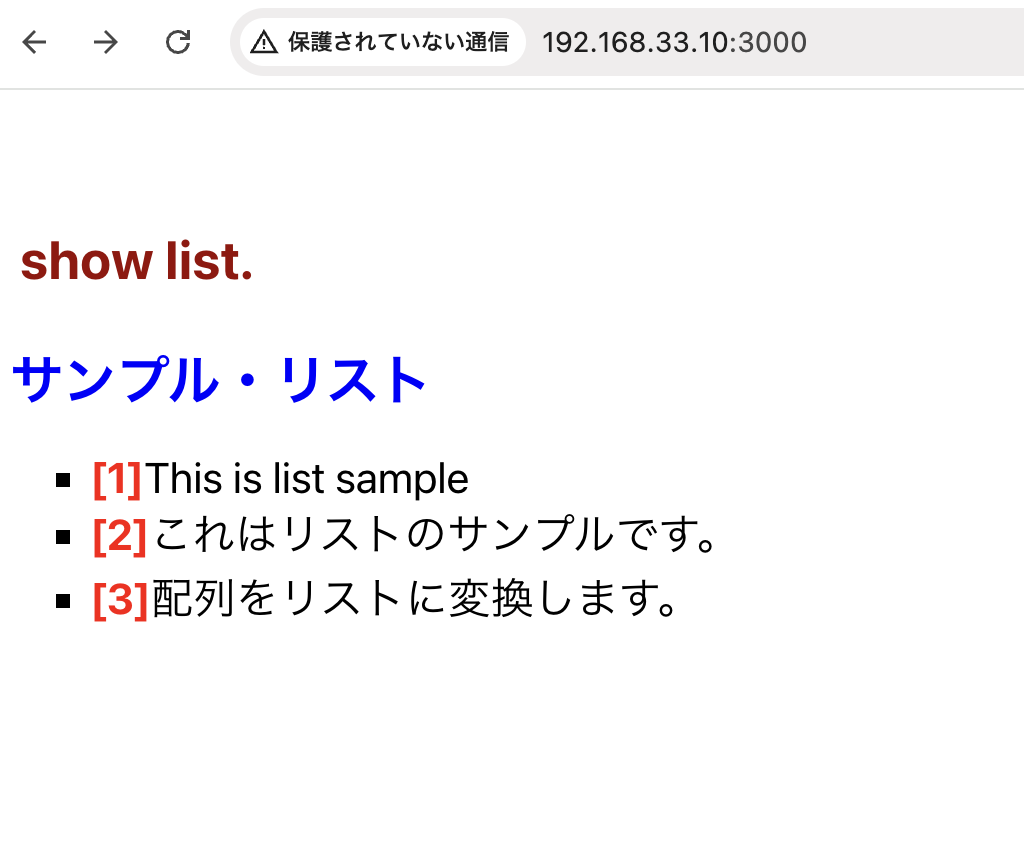
import React, {Component} from 'react';
import Rect from "./Rect";
import './App.css';
class App extends Component {
input = '';
msgStyle = {
fontSize:"20pt",
color:"#900",
margin:"20px 0px",
padding: "5px",
}
constructor(props) {
super(props);
this.state = {
message:'type your name:'
};
this.doChange = this.doChange.bind(this);
this.doSubmit = this.doSubmit.bind(this);
}
doChange(event) {
this.input = event.target.value;
}
doSubmit(event) {
this.setState({
message: 'Hello, ' + this.input + '!!'
});
event.preventDefault();
}
render() {
return <div className="App">
<h1>React</h1>
<Message title="Children">
これはコンポーネント内のコンテンツです。
マルでテキストを分割し、リストにして表示します。
改行は必要ありません。
</Message>
</div>;
}
}
class Message extends Component {
li = {
fontSize:"16pt",
color:"#06",
margin:"0px",
padding: "0px",
}
render() {
let content = this.props.children;
let arr = content.split('。');
let arr2 = [];
for(let i = 0; i < arr.length;i++){
if(arr[i].trim() != '') {
arr2.push(arr[i]);
}
}
let list = arr2.map((value,key) => (
<li style={this.li} key={key}>{value}.</li>
));
return <div>
<h2>{this.props.title}</h2>
<ol>{list}</ol>
</div>
}
}
export default App;
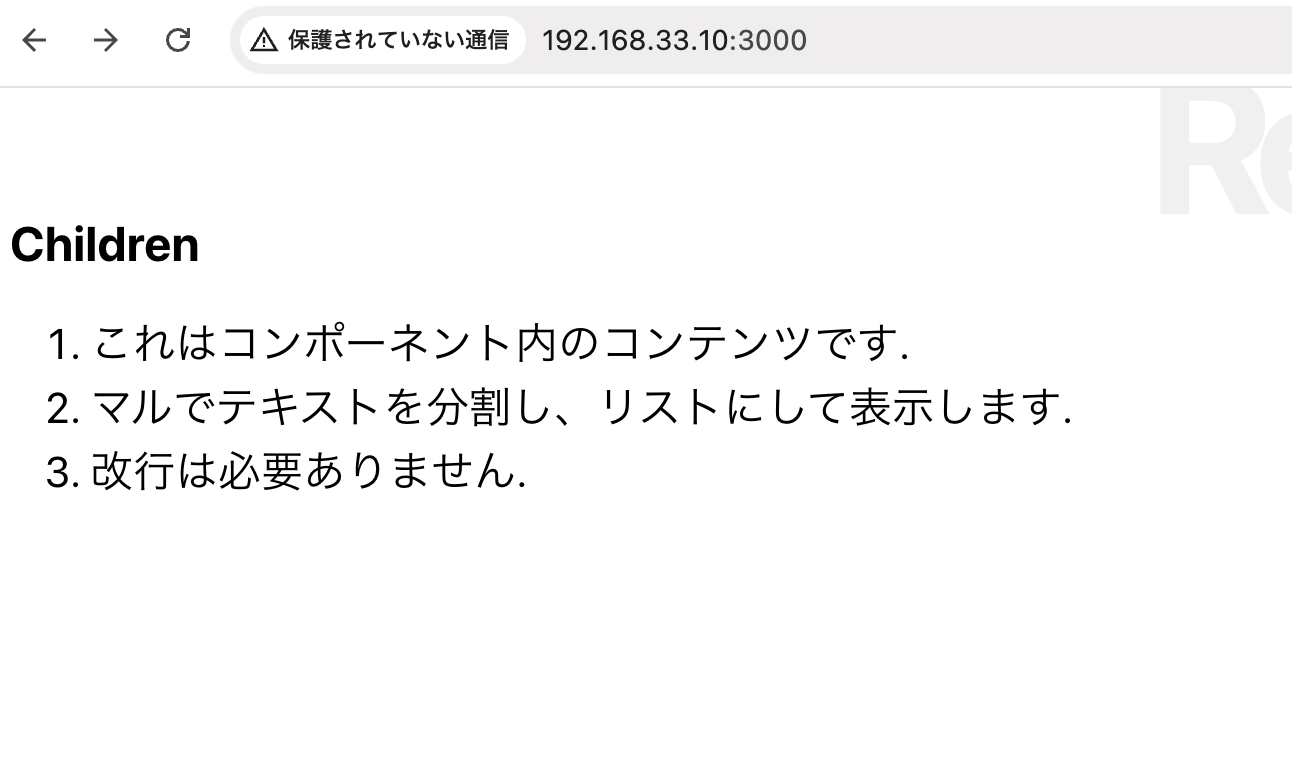