$ mkdir my-package
$ cd my-package
$ composer init
This command will guide you through creating your composer.json config.
Package name (/) [vagrant/my-package]: hpscript/my-package
Description []:
Author [hpscript <>, n to skip]:
Minimum Stability []: stable
Package Type (e.g. library, project, metapackage, composer-plugin) []: library
License []: MIT
composer.json
{
"name": "hpscript/my-package",
"type": "library",
"license": "MIT",
"minimum-stability": "stable",
"require": {}
}
$ composer require vlucas/phpdotenv
$ composer require monolog/monolog
$ composer require phpunit/phpunit –dev
$ composer require psy/psysh:@stable –dev
-> dotenvとは、getenv()メソッドや、$_ENV変数、$_SERVER変数を使って、.envというファイルから環境変数を読み込む
-> REPLは対話型評価環境
### ディレクトリの作成
$ mkdir -p src/MyPackage/Exception
$ mkdir logs
$ mkdir tests
$ mkdir docs
$ mkdir example
$ mkdir bin
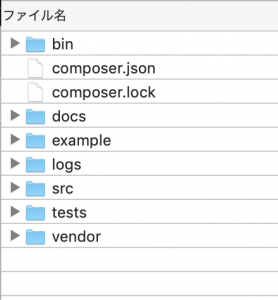
PSR-4: PHPでのファイルの読み込み・クラスのオートロードを行う仕様
composer.json
"autoload": {
"psr-4": {
"hpscript\\MyPackage\\": "src/MyPackage/"
}
}
$ composer dump-autoload
Generating autoload files
Generated autoload files
.env
TEST_NAME=hpscript
.gitignore
/vendor
.env
logs/.gitignore
*
!.gitignore
psysh.php
#!/usr/bin/env php
<?php
// 名前空間
namespace hpscript\MyPackage;
require_once __DIR__ . '/vendor/autoload.php';
$dotenv = new \Dotenv\Dotenv(__DIR__.'/');
$dotenv->load();
echo __NAMESPACE__ . " shell\n";
$sh = new \Psy\Shell();
$sh->addCode(sprintf("namespace %s;", __NAMESPACE__));
$sh->run();
echo "Bye.\n";
$ chmod +x psysh.php
phpunit.xml
<?xml version="1.0" encoding="utf-8" ?>
<phpunit colors="true" bootstrap="vendor/autoload.php">
<testsuites>
<testsuite name="All tests">
<directory>tests/</directory>
</testsuite>
</testsuites>
</phpunit>
monolog
namespace hpscript\MyPackage;
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
use Monolog\Handler\RotatingFileHandler;
class LogSample{
public static function write($message){
$log = new Logger("my-package");
$log_path = __DIR__. '/../../logs/test.log';
$handler = new StreamHandler($log_path,Logger::DEBUG);
$log->pushHandler($handler);
$rotating_handler = new RotatingFileHandler($log_path, 30, Logger::DEBUG, true);
$log->pushHandler($rotating_handler);
return $log->addDebug($message);
}
}
### phpunit
src/MyPackage/Arithmetic.php
namespace hpscript\MyPackage;
class Arithmetic {
public function add($x, $y){
return($x + $y);
}
public function subtract($x, $y){
return($x - $y);
}
public function multiply($x, $y){
return($x * $y);
}
public function divide($x, $y){
return($x / $y);
}
}
tests/ArithmeticTest.php
use PHPUnit\Framework\TestCase;
use hpscript\MyPackage\Arithmetic;
class ArithmeticTest extends TestCase {
protected $object;
protected function setUp(): void{
$this->object = new Arithmetic();
}
public function add5(){
$this->assertEquals(8, $this->object->add(3, 5));
}
public function add30(){
$this->assertEquals(45, $this->object->add(15, 30));
}
public function subtract3(){
$this->assertEquals(7, $this->object->subtract(10, 3));
}
public function subtract9(){
$this->assertEquals(-6, $this->object->subtract(3, 9));
}
public function multiply6(){
$this->assertEquals(24, $this->object->multiply(4, 6));
}
public function multiply5(){
$this->assertEquals(-20, $this->object->multiply(4, -5));
}
public function testDivide(){
$this->assertEquals(3, $this->object->divide(6, 2));
$this->assertEquals(1, $this->object->divide(6, 6));
$ ./vendor/bin/phpunit
PHPUnit 9.5.2 by Sebastian Bergmann and contributors.
. 1 / 1 (100%)
Time: 00:00.002, Memory: 6.00 MB
OK (1 test, 2 assertions)
$ php psysh.php
hpscript\MyPackage shell
Psy Shell v0.10.6 (PHP 7.4.11 — cli) by Justin Hileman
>>> $ar = new Arithmetic()
=> hpscript\MyPackage\Arithmetic {#2562}
>>> show $ar
5: class Arithmetic {
6: public function add($x, $y){
7: return($x + $y);
8: }
9:
10: public function subtract($x, $y){
11: return($x – $y);
12: }
13:
14: public function multiply($x, $y){
15: return($x * $y);
16: }
17:
18: public function divide($x, $y){
19: return($x / $y);
20: }
21: }
>>> ls $ar
Class Methods: add, divide, multiply, subtract
>>> q
Exit: Goodbye
Bye.
おお、なんか完璧には理解してないが、大まかな流れはわかった。