file_get_contents()
Want to read the whole file
readfile()
Want to output the entire contents of the file
file()
want to read line-by-line text file as an array
fopen() + fread()
Want to read the file byte by byte
SplFileObject class
want to read CSV
SplFileObject class
want to operate object-oriented
サンプルのjsonデータを使ってみます。
{
"glossary": {
"title": "example glossary",
"GlossDiv": {
"title": "S",
"GlossList": {
"GlossEntry": {
"ID": "SGML",
"SortAs": "SGML",
"GlossTerm": "Standard Generalized Markup Language",
"Acronym": "SGML",
"Abbrev": "ISO 8879:1986",
"GlossDef": {
"para": "A meta-markup language, used to create markup languages such as DocBook.",
"GlossSeeAlso": ["GML", "XML"]
},
"GlossSee": "markup"
}
}
}
}
}
$json = file_get_contents(__DIR__ . '/sample.json');
if($json === false){
echo ('file not found.');
} else {
$data = json_decode($json, true);
var_dump($data);
}
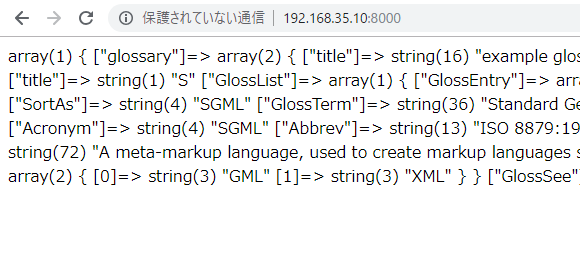
file_get_contents() は、使用頻度高いです。