内蔵サーバー
$ php ./bin/cake.php server -H 0.0.0.0 –port 8080
### View
webroot/hello.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello</title>
<style>
h1 {
font-size: 60pt;
margin: 0px 0px 10px 0px; padding: 0px 20px; color: white;
background: linear-gradient(to right, #aaa, #fff);
}
p { font-size: 14pt; color: #666; }
</style>
</head>
<body>
<header class="row">
<h1>Welcome!</h1>
</header>
<div class="row">
<p>This is sample HTML page.</p>
</div>
</body>
</html>
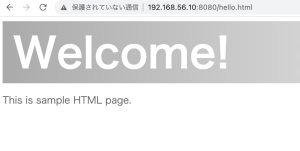
### Controller
src/Controller/HelloController.php
namespace App\Controller;
use App\Controller\AppController;
class HelloController extends AppController {
public $autoRender = false;
public function index(){
echo "<html><body><h1>Hello!</h1>";
echo "<p>This is sample page.</p></body></html>";
}
}
http://192.168.56.10:8080/hello
### クエリパラメータ
public function index(){
$id = $this->request->query['id'];
$pass = $this->request->query['pass'];
echo "<html><body><h1>Hello!</h1>";
echo "<ul><li>your id: " . $id . "</li>";
echo "<li>password: " . $pass . "</li></ul>";
echo "</body></html>";
}
http://192.168.56.10:8080/hello?id=taro&pass=yamada
パラメータなしに対応
-> if文で制御する
public function index(){
$id = "no name";
if(isset($this->request->query['id'])){
$id = $this->request->query['id'];
}
$pass = "no password";
if(isset($this->request->query['pass'])){
$id = $this->request->query['pass'];
}
echo "<html><body><h1>Hello!</h1>";
echo "<ul><li>your id: " . $id . "</li>";
echo "<li>password: " . $pass . "</li></ul>";
echo "</body></html>";
}
オブジェクトをjsonで返す
namespace App\Controller;
use App\Controller\AppController;
class HelloController extends AppController {
public $autoRender = false;
private $data = [
["name"=>"taro", "mail"=>"taro@yamada", "tel"=>"090-999-999"],
["name"=>"hanako", "mail"=>"hanako@flower", "tel"=>"080-888-888"],
["name"=>"sachiko", "mail"=>"sachiko@happy", "tel"=>"070-777-777"]
];
public function index(){
$id = 0;
if(isset($this->request->query['id'])){
$id = $this->request->query['id'];
}
echo json_encode($this->data[$id]);
}
}
なるほどー ちょっと理解した