viewでヘルパーを使用
index.html.erb
<% @posts.each do |post| %>
<li>
<%= link_to post.title, post_path(post) %>
</li>
<% end %>
posts_controller.rb
def show
@post = Post.find(params[:id])
end
show.html.erb
<h2><%= @post.title %></h2>
<p><%= @post.body %></p>
app/assets/images/logo.png
link_toでリンクを指定し、root_pathでリンク先を指定する
<div class="container">
<h1><%= link_to image_tag('logo.png', class: 'logo'), root_path %></h1>
<%= yield %>
</div>
css
.logo {
width: 200px;
height: 50px;
}
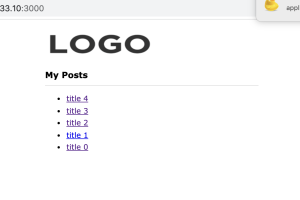
リンクを作成する
<h2>
<%= link_to 'Add New', new_post_path, class: 'header-menu' %>
My Posts
</h2>
css
.header-menu {
font-size: 12px;
font-weight: normal;
float: right;
}
controller
def new
end
def create
end
new.html.erb
<h2>Add New Post</h2>
<%= form_for :post, url:posts_path do |f| %>
<p>
<%= f.text_field:title, placeholder: 'enter title' %>
</p>
<p>
<%= f.text_area:body, placeholder: 'enter body text' %>
</p>
<p>
<%= f.submit %>
</p>
<% end %>
css
input[type="text"], textarea {
box-sizing: border-box;
width: 400px;
padding: 5px;
}
textarea {
height: 160px;
}
posts_controller.rb
def create
render plain: params[:post].inspect
end
“aa”, “body”=>”bb”} permitted: false>
def create
# render plain: params[:post].inspect
@post = Post.new(params[:post])
@post.save
redirect_to posts_path
end
private methodで書く
def create
# render plain: params[:post].inspect
@post = Post.new(post_params)
@post.save
redirect_to posts_path
end
private
def post_params
params.require(:post).permit(:title, :body)
end
バリデーション
app/models/post.rb
class Post < ApplicationRecord
validates :title, presence: true, length: {minimum: 3, message: 'too short to post!'}
validates :body, presence: true
end
controller
def create
# render plain: params[:post].inspect
@post = Post.new(post_params)
if @post.save
redirect_to posts_path
else
render 'new'
end
end
view
<p>
<%= f.text_field:title, placeholder: 'enter title' %>
<% if @post.errors.messages[:title].any? %>
<span class="error"><%= @post.errors.messages[:title][0] %></span>
<% end %>
</p>
<p>
<%= f.text_area:body, placeholder: 'enter body text' %>
<% if @post.errors.messages[:body].any? %>
<span class="error"><%= @post.errors.messages[:body][0] %></span>
<% end %>
</p>
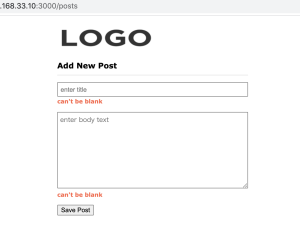
### edit
index.html.erb
<%= link_to post.title, post_path(post) %>
<%= link_to '[Edit]', edit_post_path(post), class: 'command' %>
controller
def edit
@post = Post.find(params[:id])
end
edit.html.erb
L newとほぼ同じ
<h2>Edit Post</h2>
<%= form_for @post, url:post_path(@post) do |f| %>
<p>
<%= f.text_field:title, placeholder: 'enter title' %>
<% if @post.errors.messages[:title].any? %>
<span class="error"><%= @post.errors.messages[:title][0] %></span>
<% end %>
</p>
<p>
<%= f.text_area:body, placeholder: 'enter body text' %>
<% if @post.errors.messages[:body].any? %>
<span class="error"><%= @post.errors.messages[:body][0] %></span>
<% end %>
</p>
<p>
<%= f.submit %>
</p>
<% end %>
controller
def update
@post = Post.find(params[:id])
if @post.update(post_params)
redirect_to posts_path
else
render 'edit'
end
end
改行を適切なタグに直す
<h2><%= @post.title %></h2>
<p><%= simple_format @post.body %></p>
### partialで共通部品を作る
partialはアンダーバー(_)から始める
views/posts/_form.html.erb
<%= form_for @post do |f| %>
<p>
<%= f.text_field:title, placeholder: 'enter title' %>
<% if @post.errors.messages[:title].any? %>
<span class="error"><%= @post.errors.messages[:title][0] %></span>
<% end %>
</p>
<p>
<%= f.text_area:body, placeholder: 'enter body text' %>
<% if @post.errors.messages[:body].any? %>
<span class="error"><%= @post.errors.messages[:body][0] %></span>
<% end %>
</p>
<p>
<%= f.submit %>
</p>
<% end %>
edit.html.erb
L partialの名前をアンダーバー抜きで書く
<h2>Edit Post</h2>
<%= render 'form' %>
new.html.erb
<h2>Add New Post</h2>
<%= render 'form' %>
### delete
<li>
<%= link_to post.title, post_path(post) %>
<%= link_to '[Edit]', edit_post_path(post), class: 'command' %>
<%= link_to '[x]',
post_path(post),
method: :delete,
class: 'command',
data: { confirm: 'Sure ?'} %>
</li>
controller
def destroy
@post = Post.find(params[:id])
@post.destroy
redirect_to posts_path
end