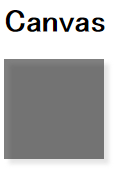
ctx.shadowColor = "#ccc";
ctx.shadowOffsetX = 5;
ctx.shadowOffsetY = 5;
ctx.shadowBlur = 5;
ctx.globalAlpha = 0.5;
ctx.fillRect(0, 0, 100, 100);
図形の変形
ctx.scale(0.8,0.8);
ctx.rotate(30/180*Math.PI);
ctx.translate(100, 10);
ctx.fillRect(0, 0, 100, 100);
stroke
ctx.beginPath();
ctx.moveTo(20, 20);
ctx.lineTo(120,20);
ctx.lineTo(120,120);
ctx.stroke();
円形・曲線:round,butt,square
ctx.beginPath();
ctx.arc(100,100,50,10/180*Math.PI,210/180*Math.PI);
ctx.lineWidth = 15;
ctx.lineCap = "round";
ctx.stroke();
テキスト描画
ctx.font = 'bold 20px Verdana';
ctx.textAlign = 'left';
ctx.fillStyle = 'red';
ctx.fillText('Google', 20, 20, 40);
ctx.strokeText('Google', 20, 120, 200);
画像表示
var img = new Image();
img.src = 'baby.jpg';
img.onload = function(){
ctx.drawImage(img, 10, 10);
}
背景パターン
var img = new Image();
img.src = 'google.png';
im.onload = function(){
var patter = ctx.createPattern(img, 'repeat');
ctx.fillStyle = pattern;
ctx.fillRect(20, 20, 100, 100);
}
設定の保存、復元
ctx.fillStyle = "yellow";
ctx.save();
ctx.fillRect(0, 0, 50, 50);
ctx.fillStyle= "blue";
ctx.fillRect(100, 0, 50, 50);
ctx.restore();
ctx.fillRect(200, 0, 50, 50);
window.onload = function(){
draw();
}
function draw(){
var canvas = document.getElementById('mycanvas');
if (!canvas || !canvas.getContext)return false;
var ctx = canvas.getContext('2d');
ctx.globalAlpha = 0.5;
for (var i = 0; i < 100; i++){
var x = Math.floor(Math.random() * 400);
var y = Math.floor(Math.random() * 200);
var r = Math.floor(Math.random() * 200);
ctx.fillStyle = "rgb("+rgb()+","+rgb()+","+rgb()+")";
ctx.beginPath();
ctx.arc(x,y,r,0,2*Math.PI);
ctx.stroke();
ctx.fill();
}
function rgb(){
return Math.floor(Math.random() * 255);
}
}