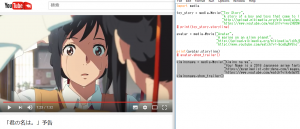
import media
toy_story = media.Movie("Toy Story",
"A story of a boy and toys that come to life",
"http://upload.wikimedia.org/wikipedia/en/1/13/Toy_Story.jpg",
"https://www.youtube.com/watch?v=vwyZH85NQC4")
# print(toy_story.storyline)
avatar = media.Movie("Avatar",
"A marine on an alien planet",
"http://upload.wikimedia.org/wikipedia/id/b/b0/Avatar-Teaser-Poster.jpg",
"http://www.youtube.com/watch?v=-9ceBgWV8io")
print(avatar.storyline)
# avatar.show_trailer()
kiminonawa = media.Movie("Kimino na wa",
"Your Name is a 2016 Japanese anime fantasy film written and directed by Makoto Shinkai",
"https://myanimelist.cdn-dena.com/images/anime/7/79999.webp",
"https://www.youtube.com/watch?v=k4xGqY5IDBE")
kiminonawa.show_trailer()
media.py
import webbrowser
class Movie():
def __init__(self, movie_title, movie_storyline, poster_image, trailer_youtube):
self.title = movie_title
self.storyline = movie_storyline
self.poster_image_url = poster_image
self.trailer_youtube_url = trailer_youtube
def show_trailer(self):
webbrowser.open(self.trailer_youtube_url)
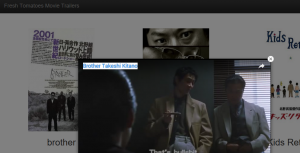
import fresh_tomatoes
import media
brother = media.Movie("brother",
"Takeshi Kitano plays Yamamoto, a lone yakuza officer. Defeated in a war with a rival family, his boss killed, he heads to Los Angeles, California.",
"https://upload.wikimedia.org/wikipedia/en/a/a1/BrotherKitano.jpg",
"https://www.youtube.com/watch?v=AgjDYR6-DTU")
# print(toy_story.storyline)
outrage = media.Movie("Outrage",
"The film begins with a sumptuous banquet at the opulent estate of the Grand Yakuza leader Sekiuchi (Soichiro Kitamura), boss of the Sanno-kai, a huge organized crime syndicate controlling the entire Kanto region, and he has invited the many Yakuza leaders under his control.",
"https://upload.wikimedia.org/wikipedia/en/f/f4/Outrage-2010-poster.png",
"https://www.youtube.com/watch?v=EXLuvZY8bMU")
#print(avatar.storyline)
# avatar.show_trailer()
#kiminonawa = media.Movie("Kimino na wa",
# "Your Name is a 2016 Japanese anime fantasy film written and directed by Makoto Shinkai",
# "https://myanimelist.cdn-dena.com/images/anime/7/79999.webp",
# "https://www.youtube.com/watch?v=k4xGqY5IDBE")
#kiminonawa.show_trailer()
kids = media.Movie("Kids Return",
"The movie is about two high school dropouts, Masaru (Ken Kaneko) and Shinji (Masanobu Ando)",
"https://upload.wikimedia.org/wikipedia/en/a/a7/KidsReturnPoster.jpg",
"https://www.youtube.com/watch?v=MvY6JCFCrQk")
ratatouille = media.Movie("Ratatouille",
"storyline",
"http://upload.wikimedia.org/wikipedia/en/5/50/RatatouillePoster.jpg",
"https://www.youtube.com/watch?v=3YG4h5GbTqU")
midnight_in_paris = media.Movie("Midnight in Paris",
"storyline",
"http://upload.wikimedia.org/wikipedia/en/9/9f/Midnight_in_Paris_Poster.jpg",
"https://www.youtube.com/watch?v=JABOZpoBYQE")
hunger_games = media.Movie("Hunger Games",
"storyline",
"http://upload.wikimedia.org/wikipedia/en/4/42/HungerGamesPoster.jpg",
"https://www.youtube.com/watch?v=PbA63a7H0bo")
movies = [brother, outrage, kids, ratatouille, midnight_in_paris, hunger_games]
fresh_tomatoes.open_movies_page(movies)
documentation
>>> import turtle
>>> turtle.Turtle.__doc__
'RawTurtle auto-creating (scrolled) canvas.\n\n When a Turtle object is created or a function derived from some\n Turtle method is called a TurtleScreen object is automatically created.\n
inheritance
class Parent():
def __init__(self, last_name, eye_color):
print("parent constructor called")
self.last_name = last_name
self.ey_color = eye_color
class Child(Parent):
def __init__(self, last_name, eye_color, number_of_toys):
print("child constructor called")
Parent.__init__(self, last_name, eye_color)
self.number_of_toys = number_of_toys
#billy_cyrus = Parent("Cyrus","blue")
#print(billy_cyrus.last_name)
myley_crus = Child("cryus", "blue", 5)
print(myley_crus.last_name)
print(myley_crus.number_of_toys)