# ディレクトリを作成します
[vagrant@localhost app]$ mkdir angel
# 作成したディレクトリに移動
[vagrant@localhost app]$ cd angel
[vagrant@localhost angel]$ ls
# composerをインストール
[vagrant@localhost angel]$ curl -sS https://getcomposer.org/installer | php
[vagrant@localhost angel]$ ls
composer.phar
# composerでlaravelをインストール。ディレクトリ名は適当にangelとしておきます。
php composer.phar create-project –prefer-dist laravel/laravel angel
# mysqlにログイン
[vagrant@localhost ~]$ mysql -u root -p
Enter password:
mysql> show databases;
# mysqlでDBの作成
mysql> create database angel;
Query OK, 1 row affected (0.12 sec)
# .envファイルを編集
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=angel
DB_USERNAME=root
DB_PASSWORD=secret
# migration 実行
[vagrant@localhost angel]$ cd angel
[vagrant@localhost angel]$ php artisan migrate
Migration table created successfully.
Migrating: 2014_10_12_000000_create_users_table
Migrated: 2014_10_12_000000_create_users_table
Migrating: 2014_10_12_100000_create_password_resets_table
Migrated: 2014_10_12_100000_create_password_resets_table
# 作成されたtable確認
mysql> use angel;
mysql> show tables;
+—————–+
| Tables_in_angel |
+—————–+
| migrations |
| password_resets |
| users |
+—————–+
3 rows in set (0.00 sec)
mysql> describe migrations;
+———–+——————+——+—–+———+—————-+
| Field | Type | Null | Key | Default | Extra |
+———–+——————+——+—–+———+—————-+
| id | int(10) unsigned | NO | PRI | NULL | auto_increment |
| migration | varchar(255) | NO | | NULL | |
| batch | int(11) | NO | | NULL | |
+———–+——————+——+—–+———+—————-+
3 rows in set (0.08 sec)
mysql> describe password_resets;
+————+————–+——+—–+———+——-+
| Field | Type | Null | Key | Default | Extra |
+————+————–+——+—–+———+——-+
| email | varchar(255) | NO | MUL | NULL | |
| token | varchar(255) | NO | | NULL | |
| created_at | timestamp | YES | | NULL | |
+————+————–+——+—–+———+——-+
3 rows in set (0.00 sec)
mysql> describe users;
+——————-+———————+——+—–+———+—————-+
| Field | Type | Null | Key | Default | Extra |
+——————-+———————+——+—–+———+—————-+
| id | bigint(20) unsigned | NO | PRI | NULL | auto_increment |
| name | varchar(255) | NO | | NULL | |
| email | varchar(255) | NO | UNI | NULL | |
| email_verified_at | timestamp | YES | | NULL | |
| password | varchar(255) | NO | | NULL | |
| remember_token | varchar(100) | YES | | NULL | |
| created_at | timestamp | YES | | NULL | |
| updated_at | timestamp | YES | | NULL | |
+——————-+———————+——+—–+———+—————-+
8 rows in set (0.00 sec)
# 認証機能を生成
[vagrant@localhost angel]$ php artisan make:auth
Authentication scaffolding generated successfully.
# routes/web.phpに追加されている
Auth::routes();
Route::get('/home', 'HomeController@index')->name('home');
# php artisan serveでサーバを立てる
[vagrant@localhost angel]$ php artisan serve –host 192.168.35.10 –port 8000
Laravel development server started:
# ${domain}/register にアクセス
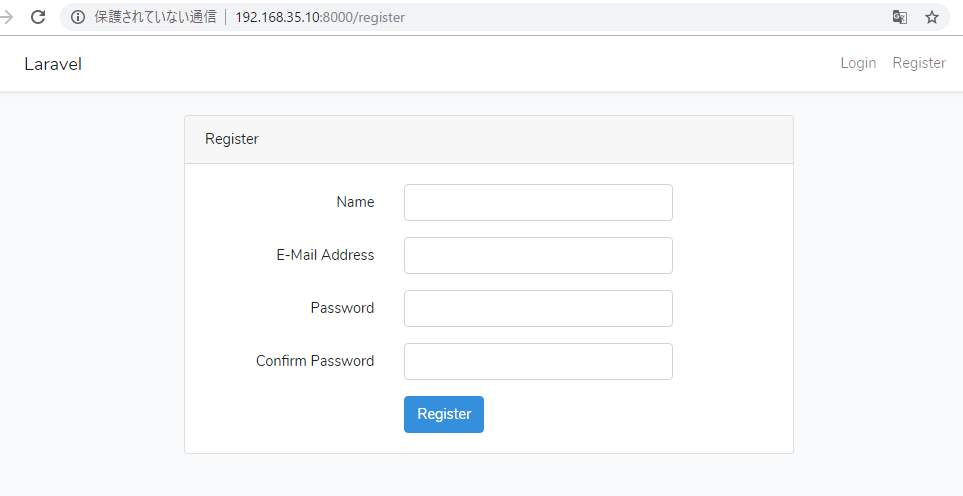
おおおおおおおおおお、これはちょっと勉強が必要だ。